Well, I take it back. I want to remember your name, and I’m terrible at names. So it helps if I have your name in front of me.
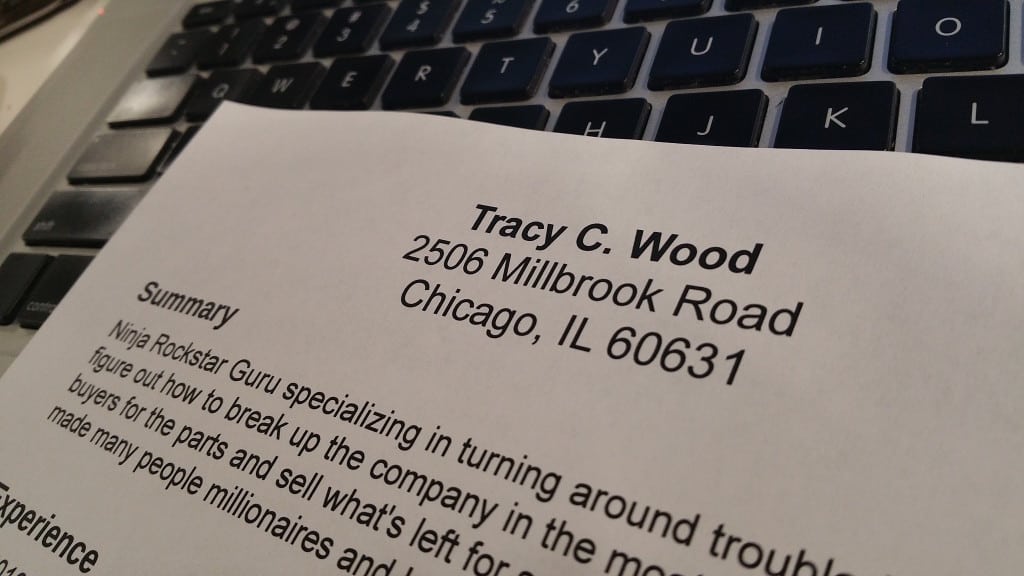
“But,” you ask, “How will you know if the candidate has the skills and experience you need?”
Good question. Let’s look at the purpose of an interview.
The Two Questions
An interview must answer two questions:
- Can the candidate do the job now and in the future?
- Will the candidate be a good fit for the team?
Neither of these questions are answered by the candidate’s resume.
Wait a minute
Unless the candidate worked for a company doing the exact work, for the exact clients you have, their experience will not tell you if they can do the current job at your company.
Put it this way: would it make sense to compare your company to another in a different industry, with different customers and a different product, and then make a judgement about performance based upon that comparison?
Company | Industry | # Customers | # Employees | Product |
---|---|---|---|---|
MyCorp | Pharmaceutical | 3 | 7 | Blue Pills |
Acme, Inc | Energy | 150 | 70 | Batteries |
Initech | Accounting Software | 3,200 | 1,500 | TPS Reports |
We will investigate the 2 questions in a future post.
Related articles
- 25 Hiring Mistakes to Avoid (smallbiztrends.com)
- Hiring is your brand: 9 small things to improve your interview process (samgerstenzang.com)
- Prevent Bad Hires With Your Hiring Process (digitalistmag.com)